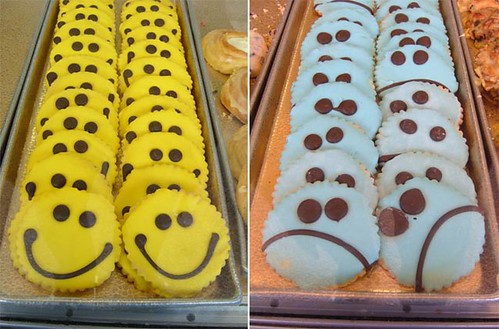
We've written a function in pseudocode. Twice. Once will be using good coding practice, care and attention. The other one won't. Because both functions will be doing the same thing, this is a great way to see the difference that good programming practice can make!
The function we've chosen is a simple one. It must take in a single (real) value, use it to evaluate two different polynomials (we'll choose a straight line and a quadratic), decide if the line value is greater than the quadratic value and return TRUE/FALSE accordingly. To make things easier, we'll assume the polynomial coefficients are defined uniquely so we can hardwire them into the code.
Let's start with the bad code...
Bad code
We'll write our code in a pseudocode, which is to say it looks like a computer language but it's more human-readable. In a real project, you'd then translate the pseudocode into the real code for whatever language you'd chosen (see here for some help with choosing a language). Most of the features of a programming language (such as FOR loops) should be obvious, and we'll use // to denote comments in the code.
FUNCTION comppoly(x)
float y1, y2
float a1=0.1, b1=0.3, a2=2.1, b2=5.3, c=0.22
y1 = a1*x + b1
y2 = a1*x^2 + b2*x + c
return(y2>y1)
END FUNCTION
We think that's not very easy to work with. And to illustrate the point, there are a couple of deliberate bugs in the above pseudocode. If you can spot them, notice that it's not very easy code in which to try to find bugs. (if you can't find them, see the bottom of the article for some clues).
Good code
Now let's look at a different way of writing the function, one that will use a lot of what we've been discussing in our other articles. Take a look and see what you think of the difference!
FUNCTION ComparePolynomials(x)
//DECLARE VARIABLES, PARAMETERS
float y_line, y_quadratic
float lineParam = [0.1, 0.3]
float quadParam = [2.1, 5.3, 0.22]
//CALCULATE THE LINE AND QUADRATIC VALUES AT X
y_line = lineParam[0]*x + lineParam[1]
y_quadratic = quadParam[0]*x^2 + quadParam[1]*x + quadParam[2]
//COMPARE THE FUNCTIONS, RETURNING A LOGICAL
return(y_line > y_quadratic)
END FUNCTION
Notice how much easier the good code is to read than the bad code. The variable names tell you what they are (as opposed to simply being "a1", "b2" etc). It's easy to see that you're multiply the correct values together and that your logical comparison is the right way round. And we only have one extra line of code (because we declared the parameters on separate lines), plus some lines of comments, which you should always include in any case.
Also notice the name of the function in each case. Right now, "comppoly" makes a reasonable amount of sense, but will you remember in a weeks' time what it stands for? How about a month? Six months? Whereas, "ComparePolynomials" will remain self-explanatory.
And remember the bugs in the bad code? In the bad code, the line beginning "y2" should use "a2" and not "a1". And the function should return "y1>y2" and not "y2>y1". Not so easy to spot, right? Whereas the same bugs in our good code would look like this.
y_quadratic = lineParam[0]*x^2 + quadParam[1]*x + quadParam[2]
return(y_quadratic > y_line)
A lot clearer!
So what's our point? Well, we have two. The first is simple: which of the above codes would you rather be working with? As you're the one writing your own code, you can choose to write good code rather than bad code! Our second point is that while writing the good code doesn't really take any longer (it's essentially the same length as the bad code, it'll save you a lot of time when you come to work with it at a later date. Our example of finding the bugs in the bad code should show you this. If you write all your code as good code, this saving can mount up enormously!
Sometimes there's no substitute for seeing good code up against (really) bad code. Which would you rather have to deal with? Then write your own code accordingly!
This makes such a good point, even to a non-specialist coder. And a great image to go with it!
ReplyDeleteHi my name is Paul Sanderson an in feb this year I started this http://www.wscoop.com its a digg style site but dedicated to web design and development
ReplyDeleteBut I need your help....
I need links to web design and development, site , articles links...... anything you think of.... also voting on topics that you think are relative and like would also be a great help.
Please are you able to help me make this into a great resource for developers and designers.
You are more than welcome to promote your own sites, projects, comments and all... at the moment the posts go straight to the font page to it is good exposer and at least some sweet backlinks
A mention or two about wscoop in you blog would do wonders too.
Thanks
Paul